Integration Process
1. Get your App Key
Sign-in to your AdGyde Dashboard, the credentials would have already been provided by AdGyde support team. In case you have not yet received the same, please contact the AdGyde Support Team.
Please follow the given steps :-
Step 1 - Visit the AdGyde Website - https://www.adgyde.com/
Step 2 - Go to console
Step 3 - Sign-in with your credentials
Step 4 - Go to Setup -> Applications from the menu option
Step 5 - Click on "Create an application" option on Top Right corner
Step 6 - Fill in the Application Name and Package Name
Step 7 - Note down the App Key for integration reference
2. Download Android SDK Please follow the given steps :-
Step 1 - Visit the AdGyde Website - https://www.adgyde.com/
Step 2 - Go to console
Step 3 - Sign-in with your credentials
Step 4 - Go to Setup -> Applications from the menu option
Step 5 - Click on "Create an application" option on Top Right corner
Step 6 - Fill in the Application Name and Package Name
Step 7 - Note down the App Key for integration reference
Please download the SDK from "Download SDK" link in top right corner of AdGyde Console.
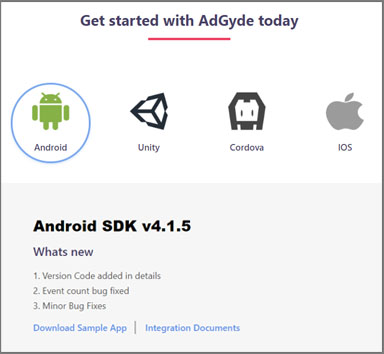
Integrate the downloaded SDK using the below steps
3. Integrate SDK into project 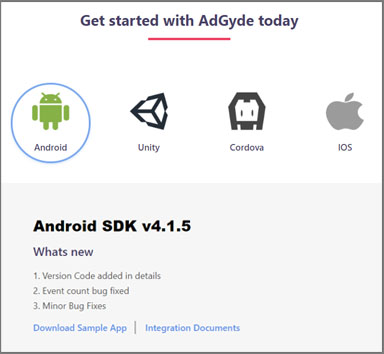
Integrate the downloaded SDK using the below steps
3.1 Add library files into your project
3.2 Follow below steps to import .aar file into your projects
3.3 Initializing AdGyde
3.6 Add Install receiver code in the androidmanifest.xml
3.8 Add dependency to project
4. Sessions - Unzip AdGyde Android SDK
- Add ADGYDE_ANDROID_SDK *.aar file to your android project
- Go to File>New>New Module
- Select "Import .JAR/.AAR Package" and click next.
- Enter the path to .aar file and click finish.
- Go to File>Project Structure (Ctrl + Shift + Alt + S).
- Under "Modules," in left menu, select "app."
- Go to "Dependencies tab.
- Click the green "+" in the upper right corner.
- Select "Module Dependency"
- Select the ADGYDE_ANDROID_SDK .AAR file from the list.
3.3 Initializing AdGyde
Android SDK needs to be initialized in the application. Please check Example project on Android SDK for complete code.
Please don't initialize the AdGyde SDK on Main Activity, this will result in incorrect session data.
Request you to create a separate application class or use any application class already present in your project. For New application class - create ExampleApplication.java and add the details under application tag in AndroidManifest.xml.
Value of android:name parameter should be full name of the newly created application class as shown in the above code snippet
3.4 Pass IMEI to AdGyde package com.adgyde.example; import android.app.Application; import android.util.Log; import com.adgyde.android.AdGyde; public class ExampleApplication extends Application implements Constants { @Override} |
Please don't initialize the AdGyde SDK on Main Activity, this will result in incorrect session data.
Request you to create a separate application class or use any application class already present in your project. For New application class - create ExampleApplication.java and add the details under application tag in AndroidManifest.xml.
<application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" </application> |
Value of android:name parameter should be full name of the newly created application class as shown in the above code snippet
If the application needs to pass the IMEI and record the same along with other user details, then Android SDK needs to be given permission to pick up IMEI explicitly. Even when application has the permission, AdGyde SDK doesn't pick up IMEI without App developer consent which user needs to pass to the SDK using AdGyde.allowPermissionIMEI(context , true) function
Along with above permission make sure application itself has the permission to pick up IMEI. Add Phone state permission in your applications manifest.xml file
Below is the sample code to initialize Android SDK with App Developer consent to pass IMEI
3.5 Embed Google Play Services into Your App Along with above permission make sure application itself has the permission to pick up IMEI. Add Phone state permission in your applications manifest.xml file
<uses-permission android:name="android.permission.READ_PHONE_STATE" /> |
Below is the sample code to initialize Android SDK with App Developer consent to pass IMEI
package com.adgyde.example; import android.app.Application; import android.util.Log; import com.adgyde.android.AdGyde; public class ExampleApplication extends Application implements Constants { @Override} |
Install the Google Play Services SDK and import it into your project. For download details, Click here.
Add the below code / entry to the AndroidManifest.xml as the last entry in the application tag (Just before </application>)
NOTE:
AdGyde recommends to always use the latest version of Google play service.
For more details see the link below.
https://developer.android.com/google/play-services/setup.html
Add the below code / entry to the AndroidManifest.xml as the last entry in the application tag (Just before </application>)
<meta-data android:name="com.google.android.gms.version" android:value="@integer/google_play_services_version"/> |
NOTE:
AdGyde recommends to always use the latest version of Google play service.
For more details see the link below.
https://developer.android.com/google/play-services/setup.html
Please refer below code
< application android:name=".ExampleApplication" <meta-data android:name="com.google.android.gms.version" <activity android:name=".MainActivity"> <intent-filter></activity><action android:name="android.intent.action.MAIN" /></intent-filter> <service android:name="com.adgyde.android.AppJobService"android:exported="true" android:permission="android.permission.BIND_JOB_SERVICE"> </service> |
3.6 Add Install receiver code in the androidmanifest.xml
Note:
Please make sure that following receiver tag is kept inside application tag.
If an application uses multiple INSTALL_REFERRER receivers, you should use com.adgyde.android.MultiInstallReceiver instead of com.adgyde.android.InstallReceiver.
MultiInstallReceiver MUST be the first receiver on Top of all the other INSTALL_REFERRER receivers
If you want to use multiple receivers, then the Manifest.xml file should look like this:
3.7 Add permissions to projectPlease make sure that following receiver tag is kept inside application tag.
<receiver android:name="com.adgyde.android.InstallReceiver" android:exported="true"> <intent-filter></receiver><action android:name="com.android.vending.INSTALL_REFERRER" /></intent-filter> |
If an application uses multiple INSTALL_REFERRER receivers, you should use com.adgyde.android.MultiInstallReceiver instead of com.adgyde.android.InstallReceiver.
MultiInstallReceiver MUST be the first receiver on Top of all the other INSTALL_REFERRER receivers
<receiver android:name="com.adgyde.android.MultiInstallReceiver" android:exported="true"> <intent-filter></receiver><action android:name="com.android.vending.INSTALL_REFERRER" /></intent-filter> |
If you want to use multiple receivers, then the Manifest.xml file should look like this:
<!--The AdGyde Install Receiver should be placed first and it will broadcast to all receivers placed below it --> <receiver android:name="com.adgyde.android.MultiInstallReceiver" android:exported="true"> <intent-filter></receiver><action android:name="com.android.vending.INSTALL_REFERRER" /></intent-filter> <!--All other receivers should be followed right after --> <receiver android:name="com.google.android.abc.AbcReceiver" android:exported="true"> <intent-filter></receiver><action android:name="com.android.vending.INSTALL_REFERRER" /></intent-filter> <receiver android:name="com.adsmobi.android.xyz.InstallReceiver" android:exported="true"> <intent-filter></receiver><action android:name="com.android.vending.INSTALL_REFERRER" /></intent-filter> |
Add following permissions to AndroidManifest.xml
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"> </usespermission> <uses-permission android:name="android.permission.ACCESS_WIFI_STATE" /> <uses-permission android:name="android.permission.INTERNET"> </uses-permission> |
3.8 Add dependency to project
Add the following dependency to Android gradle file (Module : android).
dependencies {} |
A session is a conversation between mobile application and user. A session starts with application start and it ends after the user has quit the application.
In AdGyde Android SDK, sessions are linked to the android application Life Cycle and so are calculated automatically without any integration.
5. User Flow In AdGyde Android SDK, sessions are linked to the android application Life Cycle and so are calculated automatically without any integration.
'User Flow' allows the Application Developer to gauge the movement of its users through the activities defined in the application. By analyzing the user flow Sankey diagram, the App developer can predict which activity is most popular among its users and where the drop-off rates are high.
In AdGyde Android SDK, User Flow is linked to the android application Life Cycle and so is calculated automatically without any integration.
6. Uninstall Tracking In AdGyde Android SDK, User Flow is linked to the android application Life Cycle and so is calculated automatically without any integration.
AdGyde's Uninstall Tracking functionality allows you to track the number of uninstalls for a specified application. Uninstalls is an important index which helps you to track the quality of users and hence the campaign.
Un-Install detailed integration process
7. Events Un-Install detailed integration process
AdGyde's Event Tracking allows an application owner to track the events triggered by users. What user is doing in your application are generally tracked using the events like - Registration, Add to Cart, Payment initiated, Payment.
AdGyde supports multiple types of events, please follow the below link to integrate events in your application
Events detailed integration process
8. Events AdGyde supports multiple types of events, please follow the below link to integrate events in your application
Events detailed integration process
Deep linking is the act of launching a mobile app while serving personalized content or sending the users to specific activities within the application.
Deep Linking detailed integration process
9. Demography Deep Linking detailed integration process
AdGyde demography data provides details of Age and Gender wise segregation of User, this can be used by Advertiser to target the new users and run their campaigns effectively.
Demography detailed integration process
10. Pass additional data to SDK Demography detailed integration process
AdGyde allows to pass additional data like Userid, Email and Phone Number to SDK so that same can be correlated to the AdGyde Data and logs.
- Advertiser's User id
In case Advertiser needs to relate Applications analytics data with its own User Id then advertiser can pass the same explicitly using AdGyde.setClientUserId("ADG1045984") function. Analytical data then can be shared with the install, events etc. Userid wise also.
- Email id
In case Advertiser needs to store and relate Application analytics data with the users email id then advertiser can pass the same explicitly using AdGyde.setEmail(context, "support@adgyde.com") function. Analytical data then can be shared along with email id
- Phone Number
In case Advertiser needs to store and relate Application analytics data with the users phone number then advertiser can pass the same explicitly using AdGyde.setPhoneno(context, "919876543210") function. Analytical data then can be shared along with phone number
public void onClick(View view) { } |
Menu 1
Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Menu 1
Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.